https://leetcode.com/problems/find-pivot-index/description/
Find Pivot Index - LeetCode
Can you solve this real interview question? Find Pivot Index - Given an array of integers nums, calculate the pivot index of this array. The pivot index is the index where the sum of all the numbers strictly to the left of the index is equal to the sum of
leetcode.com
누적합을 이용하는 문제였습니다.
📕 풀이방법
📔 입력 및 초기화
누적합을 저장할 vector sums와 정답을 반환할 pivotIdx를 선언 후 적절히 초기화해줍니다.
📔 풀이과정
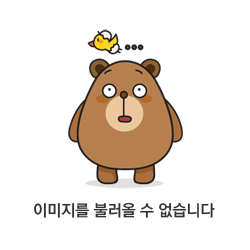
1. nums의 원소를 순회하며 왼쪽부터 i번째까지 누적합을 sums[i]에 저장해줍니다.
2. sums의 원소를 순회하며 leftSum과 rightSum을 구해줍니다. leftSum은 i번 이전까지의 누적합이므로 sums[i] - nums[i]값을 가집니다. rightSum은 nums의 모든 원소를 더한 값인 sums[nums.size() - 1]에서 i번까지의 누적합인 sums[i]를 빼준 값이 됩니다.
3. leftSum과 rightSum이 같다면 해당 index를 pivotIdx에 저장해줍니다.
📔 정답 출력 | 반환
pivotIdx를 반환합니다.
📕 Code
📔 C++
class Solution {
public:
int pivotIndex(vector<int>& nums) {
vector <int> sums(nums.size(), 0);
sums[0] = nums[0];
int pivotIdx = -1;
for(int i = 1; i < nums.size(); i++) {
sums[i] = sums[i-1] + nums[i];
}
for(int i = 0; i < sums.size(); i++) {
int leftSum = sums[i] - nums[i];
int rightSum = sums[nums.size() - 1] - sums[i];
if(leftSum == rightSum) {
pivotIdx = i;
break;
}
}
return pivotIdx;
}
};
*더 나은 내용을 위한 지적, 조언은 언제나 환영합니다.
'Algorithm > DP(Dynamic Programing)' 카테고리의 다른 글
(Python3) - 백준(BOJ) 21758 : 꿀 따기 (0) | 2024.11.13 |
---|---|
(C++) - LeetCode (easy) 746. Min Cost Climbing Stairs (0) | 2023.07.01 |
(C++) - LeetCode (easy) 509. Fibonacci Number (0) | 2023.04.11 |
(C++) - LeetCode (easy) 303. Range Sum Query - Immutable (0) | 2023.02.10 |
(C++) - LeetCode (easy) 1137. N-th Tribonacci Number (0) | 2023.01.30 |